The DS1302 is a Precision Real Time Clock (RTC) Module that keeps track of the current time and date. It is commonly used in embedded systems to maintain accurate timekeeping even when the main power supply is off. The DS1302 RTC module integrates a real-time clock/calendar and 31 bytes of static RAM.
Key Features
- Clock function: Counts seconds, minutes, hours, date, month, day of the week, and year with leap year compensation.
- RAM: 31 bytes of battery-backed static RAM for user data.
- Power: Operates on a wide voltage range of 2.0V to 5.5V.
- Battery Backup: Maintains timekeeping operation with a low-power battery backup.
- Serial Interface: Uses a simple serial interface to communicate with microcontrollers.
- Dual Power Supply: Main supply and backup supply to maintain the clock operation during power loss.
Pin Configuration
The DS1302 Real Time Clock (RTC) module typically has 6 pins:
- VCC: Main power supply (2.0V to 5.5V).
- GND: Ground.
- CLK: Serial clock input.
- DAT: Serial data input/output.
- RST: Reset (active high).
- Backup Battery: Battery backup input.
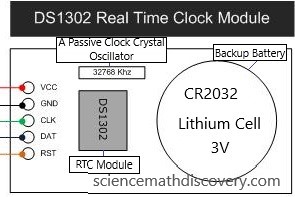
Connecting the DS1302 RTC Module to an Arduino
Components Needed
- DS1302 RTC module
- Arduino board (e.g., Uno)
- Jumper wires
- Breadboard (optional)
Wiring Diagram
- VCC to Arduino 5V
- GND to Arduino GND
- CLK to Arduino digital pin 6
- DAT to Arduino digital pin 7
- RST to Arduino digital pin 8
- Battery+ (Vbat) to the positive terminal of a 3V battery
- Battery- (GND) to the negative terminal of the 3V battery
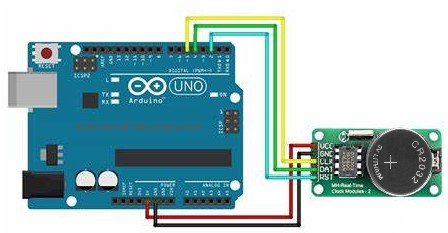
Example Arduino Code
To interface with the DS1302, you can use the DS1302RTC library available in the Arduino IDE.
Step 1: Install the DS1302RTC Library
- Open the Arduino IDE.
- Go to Sketch > Include Library > Manage Libraries.
- Search for “DS1302RTC” and install the DS1302RTC by Henning Karlsen library.
Step 2: Write the Code
Here’s an example code to read and set the time on the DS1302 RTC module:
#include <DS1302.h>
// Set the pins for the DS1302
const int RST_PIN = 8; // Reset pin
const int DAT_PIN = 7; // Data pin
const int CLK_PIN = 6; // Clock pin
DS1302 rtc(RST_PIN, DAT_PIN, CLK_PIN);
void setup() {
Serial.begin(9600);
// Initialize the RTC
rtc.halt(false);
rtc.writeProtect(false);
// Set the current time (year, month, day, hour, minute, second)
rtc.setTime(2024, 6, 4, 15, 30, 0); // Set the date and time to 2024-06-04 15:30:00
// Print the current time
Serial.print("Current Time: ");
printTime();
}
void loop() {
// Print the current time every second
delay(1000);
printTime();
}
void printTime() {
Time t = rtc.getTime();
Serial.print(t.year);
Serial.print("-");
Serial.print(t.mon);
Serial.print("-");
Serial.print(t.date);
Serial.print(" ");
Serial.print(t.hour);
Serial.print(":");
Serial.print(t.min);
Serial.print(":");
Serial.println(t.sec);
}
Explanation of the Code
- Library Inclusion and Pin Setup: The
DS1302
library is included, and the pins for the RTC module are defined. - Setup Function:
- Serial communication is initialized.
- The RTC is initialized and write protection is disabled.
- The current time is set on the RTC module.
- Loop Function: The current time is printed to the Serial Monitor every second.
- printTime Function: Reads the current time from the RTC module and prints it in a readable format.
Applications
- Clock and calendar systems
- Data logging
- Time-stamping events
- Alarms and reminders
- Power management systems
The DS1302 RTC module is an effective and reliable solution for maintaining accurate timekeeping in various electronic projects, ensuring that the correct time and date are retained even during power interruptions.
Expertise on Engineering.
Robotic and microcontroller are like toys to me.
I love playing with them.